6.1 Extract Toolset
From the docs (Esri 2020):
GIS datasets often contain more data than you need. The Extract tools let you select features and attributes in a feature class or table based on a query (SQL expression) or spatial and attribute extraction. The output features and attributes are stored in a feature class or table.
For this chapter, you will need the following R Packages:
library(arc2r)
library(sf)
library(tmap)
6.1.1 Clip
Quite often in spatial analysis, we come across cases where we do not want to use all the available data there is. In other words, we want to focus on a specific area of interest, which dictates the need for clipping the existing dataset based on it’s relationship to some other existing spatial feature. In R this operation can be easily performed using the st_intersection
function in sf
.
Let’s assume in the example below that we want to clip the available dataset of all the train stations in Switzerland by focusing our analysis on the cantons Zurich, St. Gallen, Thurgau and Aargau. In the following chunk we prerpare and visualize this situation.
# Point dataset depicting the train stations locations across Switzerland
data("haltestelle_bahn")
# Dataset depicting Switzerland on canton level
data("kantonsgebiet")
<- filter(kantonsgebiet, NAME %in% c("Zürich", "St. Gallen", "Thurgau",
kanton_filter "Aargau"))
plot(st_geometry(haltestelle_bahn))
plot(st_geometry(kanton_filter), col = "grey", add = TRUE, alpha = 1)
To clip the dataset haltestellen_bahn to the extent of _kanton_filter, we can simply use the st_intersection
function as shown below:
<- st_intersection(kanton_filter, haltestelle_bahn) haltestelle_bahn_clipped
We can now visualize the result from our operation:
plot(st_geometry(haltestelle_bahn_clipped))
plot(st_geometry(kanton_filter), add = TRUE, alpha = 1)
So, ultimately, as we can see above, the st_intersection
function creates a result where the point dataset is precisely “clipped” based on the area of interest.
The operation above produces the same outcome as the one depicted in the figure below 6.1.
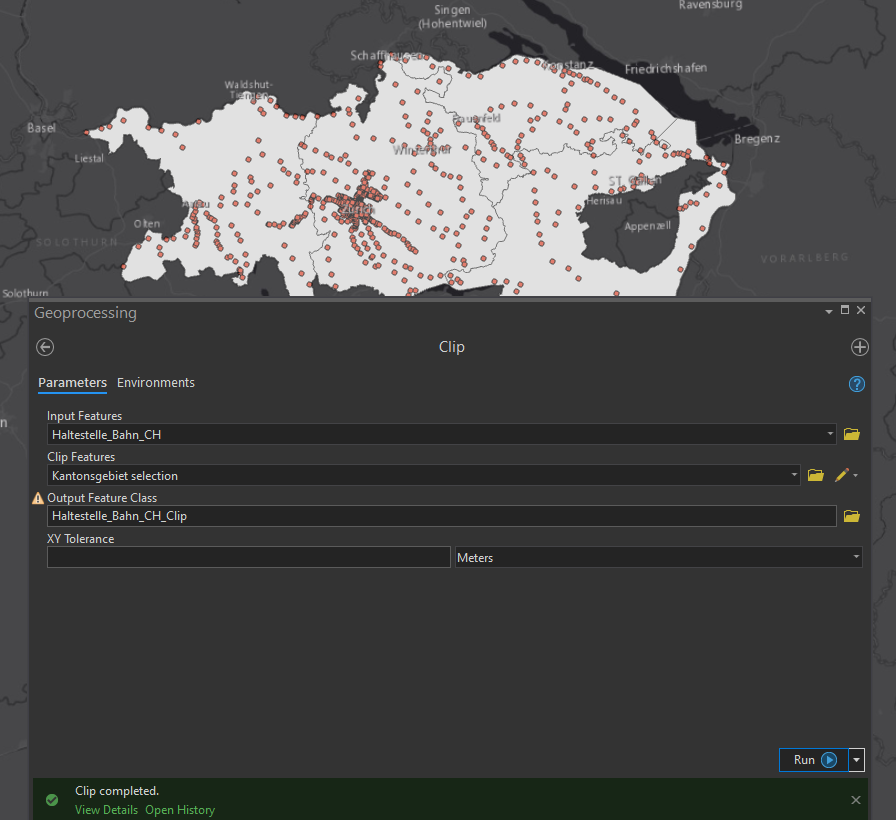
Figure 6.1: Clip operation in ArcGIS pro
6.1.2 Select
This chapter is covered in 5.1.
6.1.3 Split
The split tool is used in ArcGIS to split a dataset into multiple outputs.
When clipping the haltestellen dataset in 6.1.1 with a polygon containing multiple features (kanton_filter
). Each point in haltestelle_bahn_clipped
now has the attributes originating from kanton_filter
, which we can use to split the dataset into different parts. The function split()
(from base-R) helps us with this. It returns a list of sf
objects, that we can each save in it’s own dataset if need be.
<- split(haltestelle_bahn_clipped, haltestelle_bahn_clipped$name)
haltestellen_split
str(haltestellen_split[1:2])
## List of 2
## $ Aadorf:Classes 'sf' and 'data.frame': 1 obs. of 3 variables:
## ..$ NAME : chr "Thurgau"
## ..$ name : chr "Aadorf"
## ..$ geometry:sfc_POINT of length 1; first list element: 'XY' num [1:2] 2710374 1260730
## ..- attr(*, "sf_column")= chr "geometry"
## ..- attr(*, "agr")= Factor w/ 3 levels "constant","aggregate",..: NA NA
## .. ..- attr(*, "names")= chr [1:2] "NAME" "name"
## $ Aarau :Classes 'sf' and 'data.frame': 2 obs. of 3 variables:
## ..$ NAME : chr [1:2] "Aargau" "Aargau"
## ..$ name : chr [1:2] "Aarau" "Aarau"
## ..$ geometry:sfc_POINT of length 2; first list element: 'XY' num [1:2] 2646322 1249126
## ..- attr(*, "sf_column")= chr "geometry"
## ..- attr(*, "agr")= Factor w/ 3 levels "constant","aggregate",..: NA NA
## .. ..- attr(*, "names")= chr [1:2] "NAME" "name"
6.1.4 Split by Attributes
See 6.1.3.